· programming · 2 min read
How to pause YouTube videos dynamically with JS without swapping out the URL
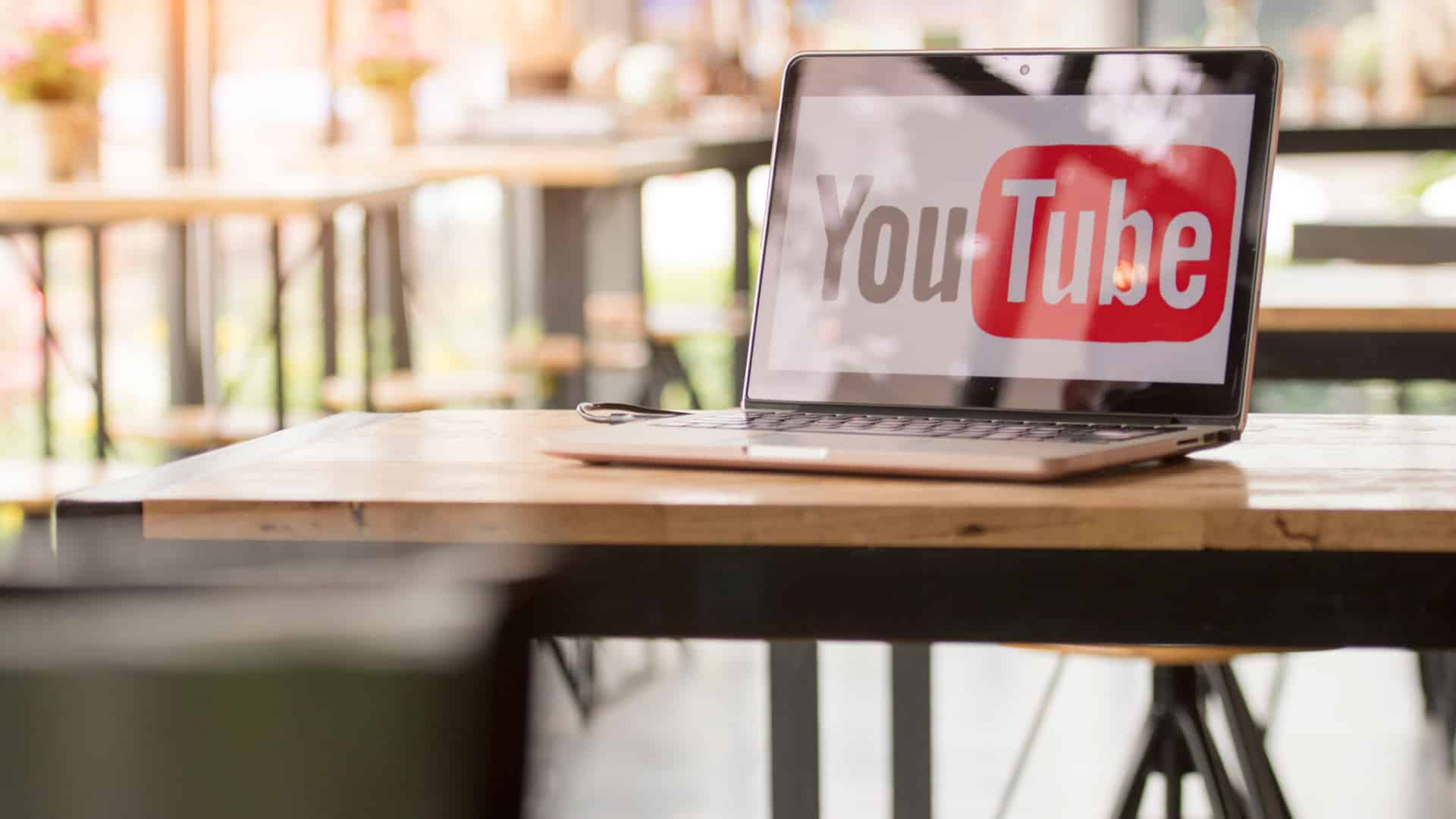
Recently I was tasked with preventing YouTube videos from continuing to play in a product detail page product image slider when you transitioned to a different slide.
Traditionally the way a developer handles this is they have an event that is triggered by some action, such as on slide change, where it finds the iframe for the video player then copies the contents of the “src” attribute into a benign attribute such as original_src” and then changes the contents of src to either empty or an image of some sort. This is then reversed when you show the element containing the video again (modal, slider, whatever).
This is problematic both because of its complexity (swapping it in and out based on whether it is shown or not) and because it could have user-end performance and bandwidth usage implications.
So I looked around for other options and found that YouTube has an API that you can control using the postMessage action on the iframe element. All you have to do is append the “enablejsapi=1” parameter to the YouTube video URL it will be ready for you to go.
The documentation about these parameters can be found here: https://developers.google.com/youtube/player_parameters
To learn more about the iframe API go here: https://developers.google.com/youtube/iframe_api_reference
The way this works is that you add the parameter to your URL when building the iframe element. Then, once the page loads set whatever action you need. In this case I am using SwiperJS on a Drupal site. So I used the “slideChange” event to trigger this. That way, whenever someone switches slides it targets all of the iframes in the slider and tells them to pause.
The command to send is `iframeElem.postMessage(’{“event”:“command”,“func”:“pauseVideo”,“args”:""}’, ’*’);`
Example Code:
// This is how you pause a YouTube video on slide change.
let $slider = new Swiper($sliderWrapper, {
on: {
slideChange: function (el) {
$('.swiper-slide').each(function () {
// Find the YouTube iframe.
var youtubePlayer = $(this).find('iframe').get(0);
if (youtubePlayer) {
// Attempt to send a command to the player to stop the playing of the video.
youtubePlayer.contentWindow.postMessage('{"event":"command","func":"pauseVideo","args":""}', '*');
}
});
}
});
For a more complete example, please checkout my gist on Github: https://gist.github.com/pthurmond/a71dec661d8b62320037b3b34453b5f3